1.Node.js“实体类”的定义//定义类Person有参数构造方法functionPerson(name,sex,age,addr,salary){this.name=name;this.sex=性别;这个。年龄=年龄;this.addr=地址;this.salary=salary;}二、定义set方法设置实体类Person的属性值//setmethodPerson.prototype.setName=function(name){this.name=name;};Person.prototype.setSex=function(sex){this.sex=sex;};Person.prototype.setAge=function(age){this.age=age;};Person.prototype.setAddr=function(addr){this.addr=addr;console.log("PersonsetAddr");};Person.prototype.setSalary=function(salary){this.salary=salary;};3.定义get方法获取实体类Person的属性值//getmethodPerson.prototype.getName=function(){returnthis.name;};Person.prototype.getSex=function(){returnthis.sex;};Person.prototype.getAge=function(){返回这个.age;};Person.prototype.getAddr=function(){返回这个.addr;};Person.prototype.getSalary=function(){返回这个.工资;};4。构造Person实例对象//使用newfull参考构造方法获取实例对象letKirito=newPerson("Kirito","Male",18,"SAO",999999999);//控制台打印Person实例console.log(Kirito);console.log("------------------------------------------------------"+"\n\n");//使用get方法获取Person属性值console.log(Kirito.getName());console.log(Kirito.getSex());console.log(Kirito.getAge());console.log(Kirito.getAddr());console.log(Kirito.getSalary());console.log("------------------------------------------------"+"\n\n");//使用新的无参结构methodtoobtaintheinstanceobjectletAusua=newPerson();//使用set方法设置Person属性值Ausua.setName("Asuna");Ausua.setSex("Female");Ausua.setAge(18);Ausua.setAddr("SAO");Ausua.setSalary(999999999);//控制台打印Person实例console.log(Ausua);console.log("--------------------------------------------------------"+"\n\n");五、Node.js实例化函数调用工作流程//Node.js实例化函数调用工作流程//letperson=newPerson();process//每个函数对象都有自己的原型对象:functionPerson()有自己的原型对象//这个原型对象是一个字典表,可以定义自己的方法:Person.prototype.set/get方法的定义//对待thisfunctionobjectasaclassandusenewtocreateainstanceoftheclass//当这个实例生成时key--->__proto__//会将函数的原型浅拷贝到实例中作为值//这样就建立了__proto__:原型关联//实例创建后绑定到这个函数的this上//在后续的函数调用过程中,这个实例是通过this传递的//this传递的实例在函数的方法体中进行一系列的初始化等操作//创建实例后,通过实例调用函数,顺序是:先查找字典表在方法体中,然后去__proto__里面找//实现在类中定义key---value和function方法后新建的实例对象也有相同的方法//我们可以对类对象进行一系列操作和属性通过调用这些函数方法Operationconsole.log("----------------------------------------------------"+"\n\n");六、Node.js继承的实现//Node.jsextendsconsole.log("inheritance");letLady=function(){};//继承方法://1.不可用//Lady.prototype=Person.prototype;//这两个原型指向同一个对象。如果PersonofLady方法被扩展,它会相应地改变。//2.在原基类的原型上浅拷贝letSuper=function(){};Super.prototype=Person.prototype;Lady.prototype=newSuper();//定义子类LadyLady的new方法。prototype.setHobby=function(hobby){this.hobby=hobby;};Lady.prototype.getHobby=function(){returnthis.hobby;};//实例化子类Lady对象ladyletlady=newLady();//设置子类Lady.setName("Illyasviel");lad??y.setSex("Female");lad??y.setAge(18);lad??y.setAddr("Fate");lad??y.setSalary(999999999);//设置子类Uniquepropertylady.setHobby("KissyouGoodBye");console.log(lady);console.log("--------------------------------------------------"+"\n\n");//3.使用util.inherits实现集成letutil=require("util");//定义子类StudentletStudent=function(cno,cname){this.cno=cno;this.cname=cname;};//子类属性对应的setget方法Student.prototype.setCno=function(cno){this.cno=cno;};Student.prototype.setCname=function(cname){this.cname=cname;};Student.prototype.getCno=function(){returnthis.cno;};Student.prototype.setCname=function(){returnthis.cname;};//继承Personutil.inherits(Student,Person);letstudent=newStudent(1,"动漫一班");student.setName("小樱");student.setSex("女");student.setAge(18);student.setAddr("命运");student.setSalary(999999999);console.log(student);console.log("-----------------------------------------------------"+"\n\n");7.Methodrewriting//methodrewritingconsole.log("methodrewriting---override");//lady原型中的setAddr函数//key:setAddr没变但是函数ofvalue:setAddrhaschanged//覆盖原来的Person.prototype.setAddrLady.prototype.setAddr=function(addr){this.hobby=addr;console.log("overridesetAddr");};lady.setAddr("命运之夜");console.log("-------------------------------------------------------"+"\n\n");//如果要调用方法重写后基类Person的setAddr,则需要显示并传递thisconsole.log("调用基类Person方法重写后的setAddr");Lady.prototype.setAddr=function(addr){Person.prototype.setAddr.call(this);};lady.setAddr("命运/零");8.总结与思考//总结与思考:////因为在Node.js等中没有Java__proto__和prototype//与java相比,两者的关系类似于继承://当一个function被创建,Node.js会自动给这个function加上prototype属性,值为空字典表对象{}//当letperson=newPerson();时,functionPerson(){}是一个构造函数(constructor)//然后JS会帮你创建构造函数的实例//该实例会继承构造函数中定义的原型所指向的所有属性和方法//该实例通过设置自己的__proto__指向来实现这种继承构造函数的原型//Node.js配合__proto__和prototype实现了原型链和对象继承//Node.js是单继承,Object.prototype是原型链的顶端,类似于Java的Object类//的constructor使用prototype来存放要共享的属性和方法,也可以设置prototype指向已有的对象来继承对象//对象的__proto__指向自己constructor的prototype//console.log(Ausua.__proto__==Person.prototype);返回真//注意:原型函数的内置属性表示修改对象原型的属性//__proto__实例对象的内置属性,JS内部使用它来查找原型链的属性//的ES规范定义了对象字面量的原型是Object.prototype//Finally//引用《JavaScript权威指南》的描述://每个JavaScript对象都有第二个JavaScript对象(或null,但很少见)与之关联。//第二个对象称为原型,第一个对象继承原型的属性。
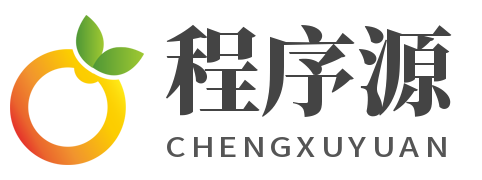