要求:学生信息由id,name,gender,admissionTime组成,可以实现学生信息的增删改查。前期准备新建一个Spring Starter Project工程添加如下依赖MySQL DriverJDBC APIMyBatis FrameworkSrping Webthymeleafapplication配置文件如下:#serverserver.port=80#close bannerspring.main.banner-mode=offspring.datasource.url=jdbc:mysql:///student?serverTimezone=GMT%2B8&characterEncoding=utf8spring.datasource.username=rootspring.datasource.password=root#spring mybatismybatis.mapper-locations=classpath:/mapper/*/*.xml#spring loglogging.level.com.cy=debug#spring thymeleafspring.thymeleaf.cache=falsespring.thymeleaf.prefix=classpath:/templates/pages/spring.thymeleaf.suffix=.html项目目录如下:封装的pojo对象为Stu.javapackage com.cy.pj.stu.pojo;import java.util.Date;public class Stu { private Long id; private String name; private String gender; private Date admissionTime; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } public Date getAdmissionTime() { return admissionTime; } public void setAdmissionTime(Date admissionTime) { this.admissionTime = admissionTime; } @Override public String toString() { return "Stu [id=" + id + ", name=" + name + ", gender=" + gender + ", admissionTime=" + admissionTime + "]"; } }Dao层接口代码为package com.cy.pj.stu.dao;import java.util.List;import org.apache.ibatis.annotations.Delete;import org.apache.ibatis.annotations.Insert;import org.apache.ibatis.annotations.Mapper;import org.apache.ibatis.annotations.Select;import org.apache.ibatis.annotations.Update;import com.cy.pj.stu.pojo.Stu;@Mapperpublic interface StuDao { //查询信息 @Select("select*from stu") List<Stu>findStu(); //删除记录 @Delete("delete from stu where id=#{id}") void deleteById(Integer id); //添加信息 @Insert("insert into stu(name,gender,admissionTime) value(#{name},#{gender},now())") void insertStu(Stu stu); //修改信息 @Select("select*from stu where id=#{id}") Stu findById(Integer id); @Update("update stu set name=#{name},gender=#{gender} where id=#{id}") void updateById(Stu stu);}Service层接口代码为package com.cy.pj.stu.service;import java.util.List;import com.cy.pj.stu.pojo.Stu;public interface StuService { List<Stu>findStu(); void deleteById(Integer id); void insertStu(Stu stu); Stu findById(Integer id); void updateById(Stu stu); }service层接口实现类代码为package com.cy.pj.stu.service.impl;import java.util.List;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.stereotype.Service;import com.cy.pj.stu.dao.StuDao;import com.cy.pj.stu.pojo.Stu;import com.cy.pj.stu.service.StuService;@Servicepublic class StuServiceImpl implements StuService { @Autowired private StuDao stuDao; @Override public List<Stu> findStu() { return stuDao.findStu(); } @Override public void deleteById(Integer id) { stuDao.deleteById(id); } @Override public void insertStu(Stu stu) { stuDao.insertStu(stu); } @Override public Stu findById(Integer id) { return stuDao.findById(id); } @Override public void updateById(Stu stu) { stuDao.updateById(stu); }}controller层StuController类代码为package com.cy.pj.stu.controller;import java.util.List;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.stereotype.Controller;import org.springframework.ui.Model;import org.springframework.web.bind.annotation.PathVariable;import org.springframework.web.bind.annotation.RequestMapping;import com.cy.pj.stu.pojo.Stu;import com.cy.pj.stu.service.StuService;@Controller@RequestMapping("/stu/")public class StuController { @Autowired private StuService stuService; @RequestMapping("doFindStu") public String doFindStu(Model model) { List<Stu> list = stuService.findStu(); model.addAttribute("stu", list); return "stu"; } @RequestMapping("doDeleteById/{id}") public String doDeleteById(@PathVariable Integer id) { stuService.deleteById(id); return "redirect:/stu/doFindStu"; } @RequestMapping("doinsert") public String doinsert() { return "stu_add"; } @RequestMapping("doInsert") public String doInsert(Stu stu) { stuService.insertStu(stu); return "redirect:/stu/doFindStu"; } @RequestMapping("doupdateById/{id}") public String doupdateById(@PathVariable Integer id,Model model) { Stu stu = stuService.findById(id); model.addAttribute("stu", stu); return "stu_mod"; } @RequestMapping("doUpdateById") public String doUpdateById(Stu stu) { stuService.updateById(stu); return "redirect:/stu/doFindStu"; } }前端主页面效果图如下实现代码为<!DOCTYPE html><html><head><meta charset="UTF-8"><title>Student Information Management System</title></head><body> <h1 align="center">Student Information Management System</h1> <h3 > <table align="center"> <thead> <tr> <th >id</th> <th >name</th> <th >gender</th> <th >admissionTime</th> <th colspan="2">operation</th> </tr> </thead> <tbody> <tr th:each="s:${stu}"> <td th:text="${s.id}">1</td> <td th:text="${s.name}">2</td> <td th:text="${s.gender}">3</td> <td th:text="${#dates.format(s.admissionTime, 'yyyy.MM.dd')}">4</td> <td> <a th:href="@{/stu/doDeleteById/{id}(id=${s.id})}">delete</a> </td> <td> <a th:href="@{/stu/doupdateById/{id}(id=${s.id})}">update</a> </td> </tr> </tbody> </table> </h3> <h2 align="center"> <a th:href="@{/stu/doinsert}">Add information</a> </h2></body></html>新增信息跳转页面如下实现代码为<!DOCTYPE html><html><head><meta charset="UTF-8"><title>Add Student Information</title></head><body> <h1 align="center">Add Student Information</h1> <form th:action="@{/stu/doInsert}" method="post"> <table align="center"> <tr> <th >name</th> <th >gender</th> </tr> <tr> <td><input type="text" name="name"></td> <td><input type="text" name="gender"></td> <td><input type="submit" value="submit"></td> </tr> </table> </form> </body></html>修改跳转页面为实现代码为<!DOCTYPE html><html><head><meta charset="UTF-8"><title>Modify Student Information</title></head><body> <h1 align="center">Modify Student Information</h1> <form th:action="@{/stu/doUpdateById}" method="post"> <input type="hidden" name="id" th:value="${stu.id}"> <table align="center"> <tr> <th >name</th> <th >gender</th> </tr> <tr> <td><input type="text" name="name" th:value="${stu.name}"></td> <td><input type="text" name="gender" th:value="${stu.gender}"></td> <td><input type="submit" value="submit"></td> </tr> </table> </form></body></html>
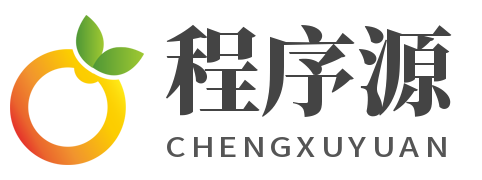