1.知道一个字符串是"hello_world_yoyo",如何得到一个队列["hello","world","yoyo"]?使用split函数对字符串进行拆分,将数据转化为列表类型:test='hello_world_yoyo'print(test.split("_"))12结果:['hello','world','yoyo']2.有一个列表["hello","world","yoyo"],如何将列表中的字符串连接起来得到字符串"hello_world_yoyo"?使用join函数将数据转成字符串:test=["hello","world","yoyo"]print("_".join(test))result:hello_world_yoyo如果不依赖joinpython提供的方法,也可以通过for循环,然后连接字符串,但是用“+”连接字符串时,结果会生成一个新的对象。使用join时,结果只是将原列表中的元素进行join,所以join效率比较高。for循环的拼接如下:test=["hello","world","yoyo"]#定义一个空字符串j=''#通过for循环打印出list中的数据foriintest:j=j+"_"+i#因为通过上面的字符串拼接得到的数据是"_hello_world_yoyo",所以前面会有一个下划线_,所以去掉下划线print(j.lstrip("_"))3.把字符串s中的每个空格替换为“%20”,输入:s="Wearehappy.",输出:"We%20are%20happy."。使用替换函数替换字符:s='Wearehappy.'print(s.replace('','%20'))12结果:We%20are%20happy.4.如何在Python中打印99乘法表?for循环打印:foriinrange(1,10):forjinrange(1,i+1):print('{}x{}={}\t'.format(j,i,i*j),end='')print()while循环实现:i=1whilei<=9:j=1whilej<=i:print("%d*%d=%-2d"%(i,j,i*j),end='')#%d:整数占位符,'-2'表示左对齐,两个占位符j+=1print()i+=1result:1x1=11x2=22x2=41x3=32x3=63x3=91x4=42x4=83x4=124x4=161x5=52x5=103x5=154x5=205x5=251x6=62x6=123x6=184x6=245x6=306x6=361x7=72x7=143x7=214x7=285x7=356x7=427x7=491x8=82x8=163x8=244x8=325x8=406x8=487x8=568x8=641x9=92x9=183x9=274x9=365x9=456x9=547x9=638x9=729x9=815。从下标0开始索引,在字符串“Hello,welcometomyworld.”中找到“welcome”,如果没有找到,返回-1。deftest():message='你好,欢迎来到我的世界。'world='welcome'ifworldinmessage:returnmessage.find(world)else:return-1print(test())结果:76.StatisticsString“你好,欢迎来到我的世界”中字母w的出现次数。deftest():message='你好,欢迎来到我的世界。'#countnum=0#forloopmessageforiinmessage:#判断'w'字符串是否在消息中,然后num+1if'w'ini:num+=1returnnumprint(test())#结果27、输入一个字符串str,输出第m个只出现n次的字符,比如在字符串gbgkkdehh中,找到第二个只出现1个字符的字符,输出结果:ddeftest(str_test,num,counts):""":paramstr_test:string:paramnum:字符串出现的次数:paramcount:字符串出现的次数:return:"""#定义一个空数组来存放逻辑处理后的数据list=[]#forloopstringdataforiinstr_test:#使用count函数统计所有字符串出现的次数count=str_test.count(i,0,len(str_test))#确定字符串出现的次数为与设置的counts个数相同,则将数据存入list数组ifcount==num:list.append(i)#返回第n次出现的字符串returnlist[counts-1]print(test('gbgkkdehh',1,2))结果:d8。判断字符串a="welcometomyworld"是否包含单词b="world",包含returnTrue,不包含returnFalse。deftest():message='welcometomyworld'world='world'ifworldinmessage:returnTruereturnFalseprint(test())结果:True9。从0开始计数,输出指定字符串deftest():message='hihowareyouhelloworld,helloyoyo!'world='hello'returnmessage.find(world)print(test())result:1510.从0开始计数,输出指定字符串A="hello"在字符串最后出现的地方B="hihowareyouhelloworld,helloyoyo!”,如果B不包含A,则输出-1。deftest(string,str):#定义last_position的初始值-1last_position=-1whileTrue:position=string.find(str,last_position+1)ifposition==-1:returnlast_positionlast_position=positionprint(test('hihowareyouhelloworld,helloyoyo!','hello'))结果:2811.给定一个数字a,判断一个数字是奇数还是偶数。whileTrue:try:#判断输入是否为整数num=int(input('Enteraninteger:'))#如果不是纯数字,需要重新输入exceptValueError:print("Theinputisnotaninteger!")continueifnum%2==0:print('偶数')else:print('奇数')break结果:输入一个整数:100个偶数12.输入一个名字判断是否姓王。deftest():user_input=input("请输入您的姓名:")ifuser_input[0]=='Wang':return"用户姓王"return"用户姓王"print(test())结果:请输入姓名:王先生用户姓王13、如何判断一个字符串是否由纯数字组成?使用Python提供的类型转换,将用户输入的数据转换为浮点数类型。如果转换抛出异常,则判断该数字不是纯数字组成的。deftest(num):try:returnfloat(num)exceptValueError:return"Pleaseenterthenumber"print(test('133w3'))14.转换字符串a="Thisisstringexample….wow!"变成大写,字符串b="WelcomeToMyWorld"变成小写。a='这是字符串示例.......哇!'b='欢迎来到我的世界'print(a.upper())print(b.lower())15.将字符串a="welcometomyworld"放在首尾空格去除Python提供了strip()方法,可以去除首尾空格,rstrip()去除尾随空格,lstrip()去除首空格,和replace("",“”)删除所有空格。a='欢迎来到我的世界'print(a.strip())也可以递归实现:deftrim(s):flag=0ifs[:1]=='':s=s[1:]flag=1ifs[-1:]=='':s=s[:-1]flag=1ifflag==1:returntrim(s)else:returnsprint(trim('Helloworld!'))通过while循环实现:deftrim(s):while(True):flag=0ifs[:1]=='':s=s[1:]flag=1ifs[-1:]=='':s=s[:-1]flag=1ifflag==0:breakreturnsprint(trim('Helloworld!'))16.Strings="ajldjlajfdljfddd",去重和小到小大排序输出“adfjl”。deftest():s='ajldjlajfdljfddd'#定义一个数组来存放数据str_list=[]#for循环s字符串中的数据,然后将数据添加到数组中foriins:#判断字符是否已经存在存在于数组字符串中,然后移除字符串并添加新字符串ifiinstr_list:str_list.remove(i)str_list.append(i)#使用sorted方法对字母进行排序a=sorted(str_list)#sorted方法returns是一个列表,这里将列表数据转换成字符串return"".join(a)print(test())result:adfjl17.打印出以下模式(菱形):deftest():n=8foriinrange(-int(n/2),int(n/2)+1):print(""*abs(i),"*"*abs(n-abs(i)*2))print(test())结果:**********************************18。给一个不超过5位的正整数(如a=12346),求出它有多少位,并把数字倒序打印出来。classTest:#计算位数deftest_num(self,num):try:#定义一个长度变量,计算数字的长度length=0whilenum!=0:#num不为0时判断,然后除法每次乘以10并四舍五入length+=1num=int(num)//10iflength>5:return"Pleaseenterthecorrectnumber"returnlengthexceptValueError:return"Pleaseenterthecorrectnumber"#ReverseorderPrintoutthesingledigitdeftest_sorted(self,num):ifself.test_num(num)!="Pleaseenterthecorrectnumber":#以相反的顺序打印出数字sorted_num=num[::-1]#返回相反的顺序单个数字的顺序returnsorted_num[-1]print(Test().test_sorted('12346'))结果:119。如果一个3位数字等于其数字的立方之和,则此数字为称为水仙花号。例如:153=13+53+33,所以153是水仙花的数字。那么如何求1000(3位数)以内的水仙花的数量。deftest():fornuminrange(100,1000):i=num//100j=num//10%10k=num%10ifi**3+j**3+k**3==num:print(str(num)+"是水仙花的数量")test()20.求出1+2+3...+100的总和。i=1forjinrange(101):i=j+iprint(i)结果:505121。计算1-2+3-4+5-…-100的值。deftest(sum_to):#定义一个初始值sum_all=0#循环你要计算的数据foriinrange(1,sum_to+1):sum_all+=i*(-1)**(1+i)返回sum_allif__name__=='__main__':result=test(sum_to=100)print(result)-5022。现有计算公式13+23+33+43+....+n3,如何实现:当输入n=5时,输出225(对应公式:13+23+33+43+53=225)。deftest(n):sum=0foriinrange(1,n+1):sum+=i*10+ireturnsumprint(test(5))result:22523已知a的值为"hello",b的值为"world",如何交换a和b的值,使得a的值为"world",b的值为"hello"?a='hello'b='world'c=aa=bb=cprint(a,b)24.如何判断一个数组是对称数组?比如[1,2,0,2,1],[1,2,3,3,2,1],这样的数组都是对称数组。从Python的角度来看,它是一个对称数组,打印的是True,而不是False。deftest():x=[1,'a',0,'2',0,'a',1]#按下标倒序比较字符串ifx==x[::-1]:returnTrue返回Falseprint(test())结果:True25。如果有一个列表a=[1,3,5,7,11],如何将它反转为[11,7,5,3,1],得到奇数位的数[1,5,11]价值?deftest():a=[1,3,5,7,11]#倒序打印数组中的数据print(a[::-1])#定义一个计数变量count=0foriina:#每次循环链表判断一条数据,计数器会+1count+=1#如果计数器是奇数,打印出来ifcount%2!=0:print(i)test()result:[11,7,5,3,1]151126。将列表a=[1,6,8,11,9,1,8,6,8,7,8]中的数字从小到大排序。a=[1,6,8,11,9,1,8,6,8,7,8]打印(排序(a))结果:[1,1,6,6,7,8,8,8,8,9,11]27。求列表L1=[1,2,3,11,2,5,3,2,5,33,88]中的最大值和最小值。L1=[1,2,3,11,2,5,3,2,5,33,88]print(max(L1))print(min(L1))result:881以上是自带的函数Python实现如下,可以自己写一个计算程序:classTest(object):def__init__(self):#测试列表数据self.L1=[1,2,3,11,2,5,3,2,5,33,88]#从列表中取第一个值,用于数据大小比较self.num=self.L1[0]deftest_small_num(self,count):""":paramcount:countis1,then表示计算最大值,为2时表示最小值:return:"""#for循环查询list中的数据foriinself.L1:ifcount==1:#循环判断数组中的数据何时高于初始值Ifi>self.num:self.num=ielifcount==2:ifi
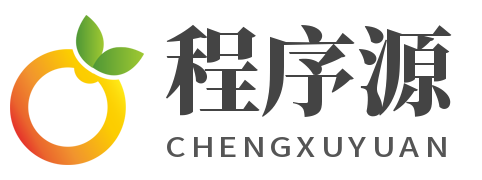