Node常用内置模块(fs模块)划分:文件操作的三个基本概念:权限位、标识符、操作符1.基本操作类别2.常用API一般分为异步操作和同步操作。这里我们使用异步操作实验(一次性文件操作,不使用buffer),Node.js有错误优先级的概念,当操作可能出错时,回调函数的第一个参数往往是错误对象constfs=要求('FS');constpath=require('path');//readFilefs.readFile(path.resolve('msg.txt'),'utf-8',(error,data)=>{if(!error)console.log(data);});//writeFile(覆盖),当路径不存在时,会创建路径并操作配置对象,可省略fs.writeFile(path.resolve('msg.txt'),'HelloNode.js',{mode:0o666,//permissionflag:'a+',//appendencoding:'utf-8'//encoding},(error)=>{if(!error)console.log('写入成功');})//apendFilefs.appendFile(path.resolve('msg.txt'),'\nHelloNode.js',{},(err)=>{if(!err)console.log('追加成功');});//copyFilefs.copyFile(path.resolve('msg.txt'),path.resolve('msg2.txt'),(err)=>{if(!err)console.log('复制成功');});//watchFilefs.watchFile(path.resolve('msg.txt'),{interval:1000},(curr,prev)=>{console.log(curr.时间);console.log(`${curr.size}${prev.size}`);})fs.appendFile(path.resolve('msg.txt'),'\nHelloNode.js',{},(err)=>{if(!err)console.log('appendsuccess22');setTimeout(()=>{fs.unwatchFile(path.resolve('msg.txt'));//unwatch},2000);});打开和关闭:fs.open(path.join(__dirname,'msg.txt'),'r',(error,fd)=>{if(!error){console.log(fd);fs.close(fd,(err)=>{if(!err)console.log('关闭成功');});}});//文件读写:constfs=require('fs');constpath=require('path');/***read从磁盘文件读取数据到内存中的buffer*fd定位当前打开的文件*buffer存储读取的数据*offset表示buffer中的写入处position*length读取缓冲区的长度*position从磁盘文件中的位置开始读取*/letbuf=Buffer.alloc(10);fs.open(path.join(__dirname,'msg.txt'),'r',(err,rfd)=>{if(err)throwerr;fs.read(rfd,buf,1,4,2,(err,readBytes,buffer)=>{console.log(readBytes);控制台日志(缓冲区);console.log(buffer.toString());})fs.close(rfd);})/***将写入数据从内存中的缓冲区写入磁盘文件*/letbuf2=Buffer.from('1234567890');fs.open('b.txt','w',(err,wfd)=>{fs.write(wfd,buf2,1,4,0??,(err,written,buffer)=>{console.log(written);console.log(buffer);控制台.log(buffer.toString());})fs.close(wfd);})包文件复制:constfs=require('fs');constpath=require('path');functionfileCopy(resFile,newFile,bufLen){让buf=Buffer.alloc(bufLen);让readOffset=0;fs.open(resFile,'r',(err,rfd)=>{if(err)throwerr;fs.open(newFile,'w',(err,wfd)=>{if(err)throwerr;functionreadAnWrite(){fs.read(rfd,buf,0,bufLen,readOffset,(err,readBytes,buffer)=>{if(!readBytes){//readBytes===0表示读取完成fs.close(rfd,()=>{});fs.close(wfd,()=>{});console.log('复制成功');返回;}readOffset+=readBytes;fs.write(wfd,buf,0,readBytes,err=>{if(err)throwerr;readAnWrite();});});}readAnWrite();})})}fileCopy(path.join(__dirname,'msg.txt'),path.join(__dirname,'b.txt'),5);3.常用操作目录APIapi:constfs=require('fs');constpath=require('path');//access判断是否有权限访问文件或目录fs.access(path.join(__dirname,'b.txt'),err=>console.log(err?err:'success'));//stat获取文件信息fs.stat(path.join(__dirname,'b.txt'),(err,stats)=>{if(!err)console.log(stats.isFile());});//mkdir创建目录fs.mkdir(path.join(__dirname,'test/jiang'),{recursive:true},err=>console.log(err?err:'makesuccess'));//rmdir删除目录fs.rmdir(path.join(__dirname,'test'),{recursive:true},err=>console.log(err?err:'dirremovesuccess'));//readdir读取目录返回文件名,目录数组fs.readdir(path.join(__dirname,'./'),(err,files)=>{if(!err)console.log(files);});//取消链接删除文件fs.unlink(path.join(__dirname,'b.txt'),err=>console.log(err?err:'文件删除成功'));目录创建的同步实现:constfs=require('fs');constpath=require('path');//access判断是否有访问文件或目录的权限fs.access(path.join(__dirname,'b.txt'),err=>console.log(err?err:'success'));//stat获取文件信息fs.stat(path.join(__dirname,'b.txt'),(err,stats)=>{if(!err)console.log(stats.isFile());});//mkdir创建目录fs.mkdir(path.join(__dirname,'test/jiang'),{recursive:true},err=>console.log(err?err:'makesuccess'));//rmdir删除目录fs.rmdir(path.join(__dirname,'test'),{recursive:true},err=>console.log(err?err:'dirremovesuccess'));//readdir读取目录并返回文件名和目录数组fs.readdir(path.join(__dirname,'./'),(err,files)=>{if(!err)console.log(files);});//取消链接删除文件fs.unlink(path.join(__dirname,'b.txt'),err=>console.log(err?err:'文件删除成功'));目录创建的异步实现:constfs=require('fs');constpath=require('路径');const{promisify}=require('util');//回调函数形式functionmkDir(dirPath,cb){letparts=dirPath.split('/');让指数=1;functionnext(){if(index>parts.length)returncb&&cb();让current=parts.slice(0,index++).join('/');fs.access(current,(err)=>{if(err){fs.mkdir(current,next);//创建目录,并使用回调函数创建下一层目录}else{next();}})}next();}mkDir('test/test2/test3',()=>{console.log('createdsuccessfully')})//处理访问和mkdir为异步/等待样式//promise形式constaccess=promisify(fs.access);constmkdir=promisify(fs.mkdir);异步函数myMkDir(dirPath,cb){letparts=dirPath.split('/');for(letindex=1;index<=parts.length;index++){让当前=parts.slice(0,index).join('/');尝试{等待访问(当前);}catch(err){awaitmkdir(current);}}cb&&cb();}myMkDir('test/test2/test3',()=>{console.log('创建成功')});异步目录删除:functionmyRemDir(dirPath,cb){fs.stat(dirPath,(err,stats)=>{if(stats.isDirectory()){//目录fs.readdir(dirPath,(err,files)=>{letdirs=files.map((item)=>path.join(dirPath,item));letindex=0;functionnext(){if(index==dirs.length){returnfs.rmdir(dirPath,cb);}else{myRemDir(dirs[index++],next);}}next();})}else{fs.unlink(dirPath,cb);}});}myRemDir(path.join(__dirname,'temp'),()=>{console.log('删除成功');})
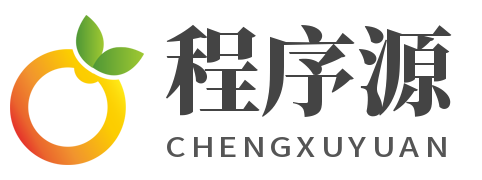